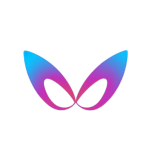
This document is intended for third-party systems to integrate with AI avatar generation capabilities.
All interfaces use Bearer Token authentication. You need to include "Authorization":"Bearer ${token}" in the request header. You can obtain the API token Here.
If the token verification fails, a 401 status code will be returned. For other exceptions, a 200 status code will be returned, and the specific exception reason can be identified through the error code in the response body.
Interface:
POST /api/v2/hifly/avatar/create_by_video
Request Parameters:
Parameter | Type | Description |
---|---|---|
title | string | Name, default "Untitled" |
video_url | url | Video URL address, choose one with file_id |
file_id | string | File ID, choose one with video_url |
Response Parameters:
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
task_id | string | Task ID |
request_id | string | Request code |
Request Example:
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/avatar/create_by_video"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
payload = {
"title": "My Digital Human",
"video_url": "https://example.com/my_video.mp4"
}
response = requests.post(url, headers=headers, json=payload)
print(response.json())
cURL Example:
curl -X POST "https://hfw-api.hifly.cc/api/v2/hifly/avatar/create_by_video" \
-H "Authorization: Bearer YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"title": "My Digital Human", "video_url": "https://example.com/my_video.mp4"}'
{
"code": 0,
"message": "",
"task_id": "1234567890123456",
"request_id": "req123456789"
}
This interface will result in point consumption.
Interface:
POST /api/v2/hifly/avatar/create_by_image
Request Parameters:
Parameter | Type | Description |
---|---|---|
title | string | Name, default "Untitled" |
image_url | url | Image URL address, choose one with file_id |
file_id | string | File ID, choose one with image_url |
model | int | Model type, 1: Video 2.0, 2: Video 2.1, default 2 |
Response Parameters:
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
task_id | string | Task ID |
request_id | string | Request code |
Request Example:
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/avatar/create_by_image"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
payload = {
"title": "My Image Avatar",
"image_url": "https://example.com/my_image.jpg",
"model": 2
}
response = requests.post(url, headers=headers, json=payload)
print(response.json())
cURL Example:
curl -X POST "https://hfw-api.hifly.cc/api/v2/hifly/avatar/create_by_image" \
-H "Authorization: Bearer YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"title": "My Image Avatar", "image_url": "https://example.com/my_image.jpg", "model": 2}'
{
"code": 0,
"message": "",
"task_id": "1234567890123456",
"request_id": "req123456789"
}
Interface:
GET /api/v2/hifly/avatar/task
Request Parameters:
Parameter | Type | Description |
---|---|---|
task_id | string | Task ID ,required |
Response Parameters:
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
status | int | Status, 1: Waiting 2: Processing 3: Completed 4: Failed |
avatar | string | Digital human identifier |
request_id | string | Request code |
Request Example:
Python Example:
import requests
task_id = "1234567890123456"
url = f"https://hfw-api.hifly.cc/api/v2/hifly/avatar/task?task_id={task_id}"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
response = requests.get(url, headers=headers)
print(response.json())
cURL Example:
curl -X GET "https://hfw-api.hifly.cc/api/v2/hifly/avatar/task?task_id=1234567890123456" \
-H "Authorization: Bearer YOUR_TOKEN"
Response Example:
{
"code": 0,
"message": "",
"status": 3,
"avatar": "av_abc123xyz",
"request_id": "req123456789"
}
POST /api/v2/hifly/voice/create
Parameter | Type | Description |
---|---|---|
title | string | Voice name, required, no more than 20 characters |
voice_type | int | Voice type, required, 8: Basic version of voice cloning v2, currently only supports 8 |
audio_url | url | Voice file URL, supports mp3, m4a, wav formats, within 20M, duration range 5 seconds to 3 minutes. Choose one with file_id |
file_id | string | Audio file ID, choose one with audio_url |
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
task_id | string | Task ID |
request_id | string | Request code |
Request Example:
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/voice/create"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
payload = {
"title": "My Voice",
"voice_type": 8,
"audio_url": "https://example.com/my_audio.mp3"
}
response = requests.post(url, headers=headers, json=payload)
print(response.json())
cURL Example:
curl -X POST "https://hfw-api.hifly.cc/api/v2/hifly/voice/create" \
-H "Authorization: Bearer YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"title": "My Voice", "voice_type": 8, "audio_url": "https://example.com/my_audio.mp3"}'
Response Example:
{
"code": 0,
"message": "",
"task_id": "1234567890123456",
"request_id": "req123456789"
}
POST /api/v2/hifly/voice/edit
Parameter | Type | Description |
---|---|---|
voice | string | Voice identifier, required |
rate | string | Speech rate, required, value between 0.5 and 2.0, default 1.0, string format |
volume | string | Volume, required, value between 0.1 and 2.0, default 1.0, string format |
pitch | string | Pitch, required, value between 0.1 and 2.0, default 1.0, string format |
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
request_id | string | Request code |
Request Example:
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/voice/edit"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
payload = {
"voice": "voice_abc123",
"rate": "1.2",
"volume": "1.0",
"pitch": "0.9"
}
response = requests.post(url, headers=headers, json=payload)
print(response.json())
cURL Example:
curl -X POST "https://hfw-api.hifly.cc/api/v2/hifly/voice/edit" \
-H "Authorization: Bearer YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"voice": "voice_abc123", "rate": "1.2", "volume": "1.0", "pitch": "0.9"}'
Response Example:
{
"code": 0,
"message": "",
"request_id": "req123456789"
}
Query completed cloned voices and public voices
GET /api/v2/hifly/voice/list
Parameter | Type | Description |
---|---|---|
page | int | Current page, default 1 |
size | int | Number per page, default 20 |
kind | int | Voice category, 1: Self-cloned, 2: Public voice, default 1 |
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
data | array | Voice array |
voice |
string | Voice identifier |
type |
int | Voice type, 10: Public voice, 20: High-fidelity voice |
title |
string | Voice name |
rate |
string | Speech rate |
volume |
string | Volume |
pitch |
string | Pitch |
request_id | string | Request code |
Request Example:
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/voice/list"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
params = {
"page": 1,
"size": 10,
"kind": 1
}
response = requests.get(url, headers=headers, params=params)
print(response.json())
cURL Example:
curl -X GET "https://hfw-api.hifly.cc/api/v2/hifly/voice/list?page=1&size=10&kind=1" \
-H "Authorization: Bearer YOUR_TOKEN"
Response Example:
{
"code": 0,
"message": "",
"data": [
{
"voice": "voice_abc123",
"type": 20,
"title": "My Voice",
"rate": "1.0",
"volume": "1.0",
"pitch": "1.0"
},
{
"voice": "voice_def456",
"type": 10,
"title": "Public Voice 1",
"rate": "1.0",
"volume": "1.0",
"pitch": "1.0"
}
],
"request_id": "req123456789"
}
Interface:
GET /api/v2/hifly/voice/task
Request Parameters:
Parameter | Type | Description |
---|---|---|
task_id | string | Task ID |
Response Parameters:
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
status | int | Status, 1: Waiting 2: Processing 3: Completed 4: Failed |
voice | string | Voice identifier |
demo_url | url | Demo voice file address |
request_id | string | Request code |
Request Example:
Python Example:
import requests
task_id = "1234567890123456"
url = f"https://hfw-api.hifly.cc/api/v2/hifly/voice/task?task_id={task_id}"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
response = requests.get(url, headers=headers)
print(response.json())
cURL Example:
curl -X GET "https://hfw-api.hifly.cc/api/v2/hifly/voice/task?task_id=1234567890123456" \
-H "Authorization: Bearer YOUR_TOKEN"
Response Example:
{
"code": 0,
"message": "",
"status": 3,
"voice": "voice_abc123",
"demo_url": "https://example.com/demo_audio.mp3",
"request_id": "req123456789"
}
This interface will result in point consumption.
Interface:
POST /api/v2/hifly/video/create_by_audio
Request Parameters
Parameter | Type | Description |
---|---|---|
audio_url | url | Audio file address, required for audio-driven, supports mp3, m4a, wav formats, within 100M, duration range 5 seconds to 30 minutes |
file_id | string | Audio file ID |
avatar | string | Digital human identifier |
title | string | Work name, default "Untitled", no more than 20 characters. |
pipeline | string | Model type, default 1.5 |
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
task_id | string | Task ID |
request_id | string | Request code |
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/video/create_by_audio"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
payload = {
"title": "Audio Driven Video",
"audio_url": "https://example.com/my_audio.mp3",
"avatar": "av_abc123xyz",
"pipeline": "1.5"
}
response = requests.post(url, headers=headers, json=payload)
print(response.json())
cURL Example:
curl -X POST "https://hfw-api.hifly.cc/api/v2/hifly/video/create_by_audio" \
-H "Authorization: Bearer YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"title": "Audio Driven Video", "audio_url": "https://example.com/my_audio.mp3", "avatar": "av_abc123xyz", "pipeline": "1.5"}'
Response Example:
{
"code": 0,
"message": "",
"task_id": "1234567890123456",
"request_id": "req123456789"
}
This interface will result in point consumption.
Interface:
POST /api/v2/hifly/video/create_by_tts
Request Parameters:
Parameter | Type | Description |
---|---|---|
voice | string | Voice identifier, required for text-driven/output audio only, refer to Voice Cloning, or obtain public voice through Voice List |
text | string | Text content, required for text-driven/output audio only, no more than 10000 characters, HTML tags not supported |
avatar | string | Digital human identifier |
title | string | Work name, default "Untitled", no more than 20 characters. |
pipeline | string | Model type, default 1.5 |
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
task_id | string | Task ID |
request_id | string | Request code |
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/video/create_by_tts"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
payload = {
"title": "Text Driven Video",
"text": "This is a test text for generating digital human video.",
"voice": "voice_abc123",
"avatar": "av_abc123xyz",
"pipeline": "1.5"
}
response = requests.post(url, headers=headers, json=payload)
print(response.json())
cURL Example:
curl -X POST "https://hfw-api.hifly.cc/api/v2/hifly/video/create_by_tts" \
-H "Authorization: Bearer YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"title": "Text Driven Video", "text": "This is a test text for generating digital human video.", "voice": "voice_abc123", "avatar": "av_abc123xyz", "pipeline": "1.5"}'
Response Example:
{
"code": 0,
"message": "",
"task_id": "1234567890123456",
"request_id": "req123456789"
}
This interface will result in point consumption.
Interface:
POST /api/v2/hifly/audio/create_by_tts
Request Parameters:
Parameter | Type | Description |
---|---|---|
voice | string | Voice identifier, required for text-driven/output audio only, refer to Voice Cloning, or obtain public voice through Voice List |
text | string | Text content, required for text-driven/output audio only, no more than 10000 characters, HTML tags not supported |
title | string | Work name, default "Untitled", no more than 20 characters. |
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
task_id | string | Task ID |
request_id | string | Request code |
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/audio/create_by_tts"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
payload = {
"title": "Audio Creation",
"text": "This is a test text for generating audio.",
"voice": "voice_abc123"
}
response = requests.post(url, headers=headers, json=payload)
print(response.json())
cURL Example:
curl -X POST "https://hfw-api.hifly.cc/api/v2/hifly/audio/create_by_tts" \
-H "Authorization: Bearer YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"title": "Audio Creation", "text": "This is a test text for generating audio.", "voice": "voice_abc123"}'
Response Example:
{
"code": 0,
"message": "",
"task_id": "1234567890123456",
"request_id": "req123456789"
}
Interface:
GET /api/v2/hifly/video/task
Request Parameters:
Parameter | Type | Description |
---|---|---|
task_id | string | Task ID |
Response Parameters:
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
status | int | Work status, 1: Waiting 2: Processing 3: Completed 4: Failed |
video_Url | url | Synthesized video address. This is a temporary address, please save it as soon as possible. The URL will contain query parameters, please ensure compatibility when downloading the video. |
duration | int | Work duration, unit: seconds |
request_id | string | Request code |
Request Example:
Python Example:
import requests
task_id = "1234567890123456"
url = f"https://hfw-api.hifly.cc/api/v2/hifly/video/task?task_id={task_id}"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
response = requests.get(url, headers=headers)
print(response.json())
cURL Example:
curl -X GET "https://hfw-api.hifly.cc/api/v2/hifly/video/task?task_id=1234567890123456" \
-H "Authorization: Bearer YOUR_TOKEN"
Response Example:
{
"code": 0,
"message": "",
"status": 3,
"video_Url": "https://example.com/videos/abc123.mp4?token=xyz789",
"duration": 45,
"request_id": "req123456789"
}
After the task is completed, the message will be actively pushed to the callback address.
If the response status code is not 200, it is considered a notification failure, and it will retry within 5 minutes, and will not retry thereafter.
You can set the callback address Here.
Request Parameters
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
type | int | Message type: 2 for digital human |
status | int | Status, 3: Completed 4: Failed |
avatar | string | Digital human identifier |
Response Example:
{
"message": "",
"code": 0,
"type": 2,
"status": 3,
"avatar": "av_abc123xyz"
}
Request Parameters
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
type | int | Message type: 3 for voice |
status | int | Status, 3: Completed 4: Failed |
voice | string | Voice identifier |
demo_url | url | Demo voice file address |
Response Example:
{
"message": "",
"code": 0,
"type": 3,
"status": 3,
"voice": "voice_abc123",
"demo_url": "https://example.com/demo_audio.mp3"
}
Request Parameters
Parameter | Type | Description |
---|---|---|
status | int | Work status, 3: Completed 4: Failed |
video_Url | url | Synthesized video address. This is a temporary address, please save it as soon as possible. The URL will contain query parameters, please ensure compatibility when downloading the video. |
type | int | Message type: 1 for work. |
duration | int | Work duration, unit: seconds |
message | string | Error message on failure |
code | int | Error code on failure |
title | string | Work name |
Response Example:
{
"status": 3,
"video_Url": "https://example.com/videos/abc123.mp4?token=xyz789",
"type": 1,
"duration": 45,
"message": "",
"code": 0,
"title": "My Video Creation"
}
First obtain the upload address, then upload the file
Interface:
POST /api/v2/hifly/tool/create_upload_url
Request Parameters
Parameter | Type | Description |
---|---|---|
file_extension | string | Extension, such as mp4, mp3, etc. |
Response Parameters
Parameter | Type | Description |
---|---|---|
upload_url | string | Upload address |
content_type | string | File mime-type, needs to set Content-Type when uploading |
file_id | string | File ID |
request_id | string | Request code |
Request Example:
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/tool/create_upload_url"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
payload = {
"file_extension": "mp4"
}
response = requests.post(url, headers=headers, json=payload)
print(response.json())
cURL Example:
curl -X POST "https://hfw-api.hifly.cc/api/v2/hifly/tool/create_upload_url" \
-H "Authorization: Bearer YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"file_extension": "mp4"}'
Response Example:
{
"upload_url": "https://upload.hifly.cc/test%2Fa.mp4?Expires=1745576446&OSSAccessKeyId=LTAI5tPx2KRcdE3ihKTp5c36&Signature=rY86EuJEqzwYkOPnwOJDHPwP9Ew%3D",
"content_type": "video/mp4",
"file_id": "file_abc123xyz",
"request_id": "req123456789"
}
Description
Example
Python Example:
import requests
# Assuming we have obtained the upload address from the previous step
upload_url = "https://upload.hifly.cc/test%2Fa.mp4?Expires=1745576446&OSSAccessKeyId=LTAI5tPx2KRcdE3ihKTp5c36&Signature=rY86EuJEqzwYkOPnwOJDHPwP9Ew%3D"
content_type = "video/mp4"
file_path = "/path/to/your/file.mp4"
with open(file_path, 'rb') as file:
headers = {
"Content-Type": content_type
}
response = requests.put(upload_url, headers=headers, data=file)
print(f"Status code: {response.status_code}")
cURL Example:
curl -i -X PUT -T ./my_video.mp4 \
-H 'Content-Type: video/mp4' \
'https://upload.hifly.cc/test%2Fa.mp4?Expires=1745576446&OSSAccessKeyId=LTAI5tPx2KRcdE3ihKTp5c36&Signature=rY86EuJEqzwYkOPnwOJDHPwP9Ew%3D'
GET /api/v2/hifly/account/credit
None
Parameter | Type | Description |
---|---|---|
message | string | Error message on failure |
code | int | Error code on failure |
left | int | Credit balance |
request_id | string | Request code |
Request Example:
Python Example:
import requests
url = "https://hfw-api.hifly.cc/api/v2/hifly/account/credit"
headers = {
"Authorization": "Bearer YOUR_TOKEN"
}
response = requests.get(url, headers=headers)
print(response.json())
cURL Example:
curl -X GET "https://hfw-api.hifly.cc/api/v2/hifly/account/credit" \
-H "Authorization: Bearer YOUR_TOKEN"
Response Example:
{
"code": 0,
"message": "",
"left": 10000,
"request_id": "req123456789"
}
Value | Description |
---|---|
11 | Incorrect parameter |
14 | Resource not found |
1001 | The number of works being generated has reached the limit |
1002 | Insufficient credits |
1005 | Open membership |
1006 | Membership level is not enough |
1009 | High-fidelity voice is sold out |
1011 | Voice is similar to a celebrity |
1013 | The number of high-fidelity voice clones has reached the limit |
1015 | The number of submitted works has reached the limit |
2003 | Invalid Token |
2011 | File size exceeds the limit |
2012 | File type not supported |
2013 | Failed to obtain audio resource |
2014 | Failed to obtain video resource |
2015 | Digital human cloning failed |
2016 | Failed to obtain image resource |